In previous posts, we assembled remote temperature stations and validated that the microcontroller functioned and connected to wifi.
Priority #2: Accessing Sensor Data
Each sensor you connect to a microcontroller will likely require slightly different access methods and code. Fear not, however. Unless you’re working on the electronic frontier in a lab or with a home-brewed sensor, someone has been there first and likely wrote a library for you to use. Our DS18B20 temperature sensors require two – OneWire by Jim Studt et. al. and DallasTemperature by Miles Burton and friends. OneWire abstracts accessing multiple sensors on one bus and DallasTemperature was specifically written for the DS18B20 temperature sensors and to smooth out some of OneWire’s rough edges.
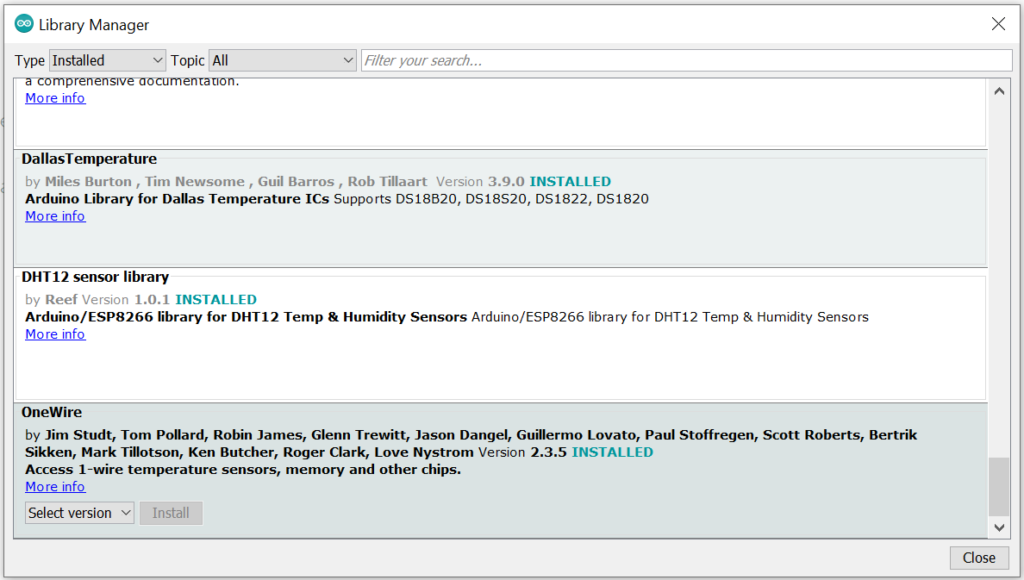
#include <OneWire.h>
#include <DallasTemperature.h>
Next, we declare some instance variables and chain them together. The OneWire constructor needs to know which GPIO pin to access for temperature data and the DallasTemperature sensor constructor accepts the OneWire instance.
// GPIO where the DS18B20 is connected to
const int oneWireBus = 13;
// Setup a oneWire instance to communicate with any OneWire devices
OneWire oneWire(oneWireBus);
// Pass our oneWire reference to Dallas Temperature sensor
DallasTemperature sensors(&oneWire);
Next, the ESP32 needs to set GPIO pin 13 to the proper state. I found (though much hair-pulling frustration) that the ESP32-CAM wifi hardware shares some interest in pin 13. To deconflict its use, we set it to OUTPUT initially. Kind of like NULL (take that, hardware!). After the wifi is configured, we set pin 13 to INPUT so DallasTemperature can read the temperature data. Finally, tell the DallasTemperature instance
void setup() {
pinMode(oneWireBus, OUTPUT);
connectToWifi();
pinMode(oneWireBus, INPUT);
// Start the DS18B20 sensor
sensors.begin();
}
“Get to the part where we measure temperatures, already!” Right. All you do is … request the temperature from DallasTemperature. Well, first you tell the instance to communicate with the sensor. Once that’s complete, pull the current temperature in your desired format (not sure if Kelvin is an option…).
float getTempValueF(){
sensors.requestTemperatures();
return sensors.getTempFByIndex(0);
}
float getTempValueC(){
sensors.requestTemperatures();
return sensors.getTempFByIndex(0);
}
Now we’re rolling in the temperatures, baby!
My code is publically available on my TempBuddy GitHub repository.